3.1.1. Sending Push Notifications Using AppDelegate
Setup guide with Mindbox’s AppDelegate
Make sure the following steps have been completed successfully:
By the end of this guide, Mindbox will be able to send push notifications that will be displayed on the target device(s).
To check that your push notifications are sent correctly, please refer to this guide on how to check that push notifications are sent.
Push notifications are messages with a header, body text, and URL which opens when the user clicks the notification. Unlike rich push notifications, standard ones lack buttons and images.
1. Enable push notifications in app settings
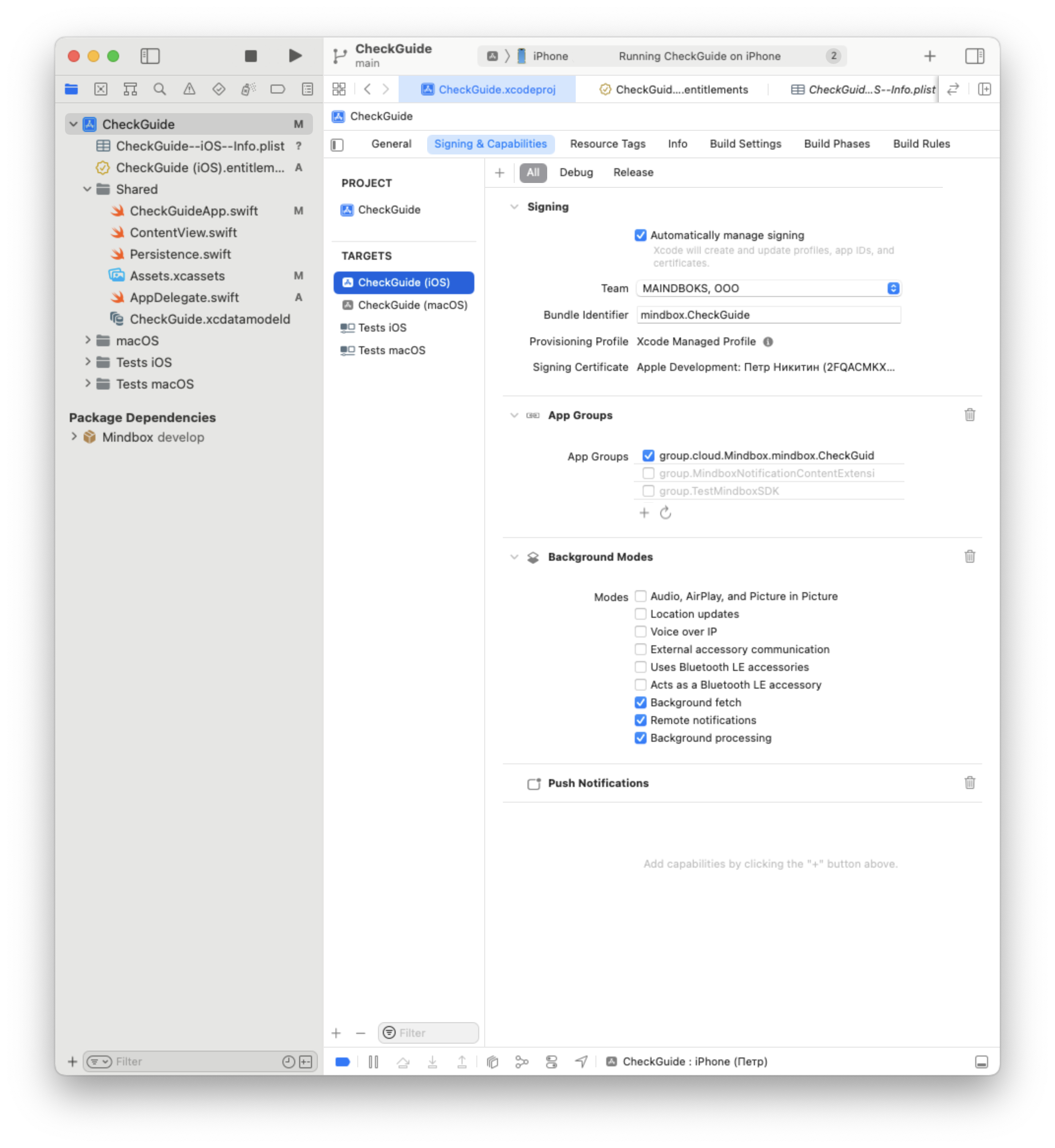
- Open project settings;
- Select your main target;
- Open the
Signing & Capabilities
tab; - Click Add and select
Push Notifications
andBackground modes
; - In the
Background Modes
section, select:- Background fetch;
- Remote notifications;
- Background processing.
Pass the keys to the project manager to connect to Apple Push Notification service or add the keys manually.
2. Request permission to display notifications
Open AppDelegate.swift
to find and implement the registerForRemoteNotifications
function. Call Mindbox.shared.notificationsRequestAuthorization
from this function.
To call the function, use the didFinishLaunchingWithOptions method
.
//
// AppDelegate.swift
// Sample
//
// Created by Peter Nikitin on 06.04.2022.
//
import Foundation
import Mindbox
import UIKit
class AppDelegate: UIResponder, UIApplicationDelegate {
...
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
//Call the notification registration method
registerForRemoteNotifications()
...
}
// MARK: registerForRemoteNotifications
// Function to request permission to display notifications. In the Completion block, send the permission status to Mindbox’s SDK
func registerForRemoteNotifications() {
UNUserNotificationCenter.current().delegate = self
DispatchQueue.main.async {
UIApplication.shared.registerForRemoteNotifications()
UNUserNotificationCenter.current().requestAuthorization(options: [ .alert, .sound, .badge]) { granted, error in
print("Permission granted: \(granted)")
if let error = error {
print("NotificationsRequestAuthorization failed with error: \(error.localizedDescription)")
}
Mindbox.shared.notificationsRequestAuthorization(granted: granted)
}
}
}
...
}
3. Ensure standard notifications will be displayed on recipients’ screens
Open AppDelegate.swift
to find and enable the userNotificationCenter.willPresent
function for notifications to be displayed on screens.
//
// AppDelegate.swift
// Sample
//
// Created by Peter Nikitin on 06.04.2022.
//
import Foundation
import Mindbox
import UIKit
class AppDelegate: UIResponder, UIApplicationDelegate {
...
func userNotificationCenter(
_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void
) {
completionHandler([.alert, .badge, .sound])
}
...
}
4. Inherit AppDelegate from MindboxAppDelegate
Inherit AppDelegate
from MindboxAppDelegate
. This class has some important methods built in, so you will only have to initialize the libraries and sign up for notifications.
import Mindbox
//Before: AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate
class AppDelegate: MindboxAppDelegate {
// Call super when using MindboxAppDelegate
super.application(application, didFinishLaunchingWithOptions: launchOptions)
// ....
}
To set up all your API methods without inheriting them, please refer to this guide.
Updated about 1 year ago